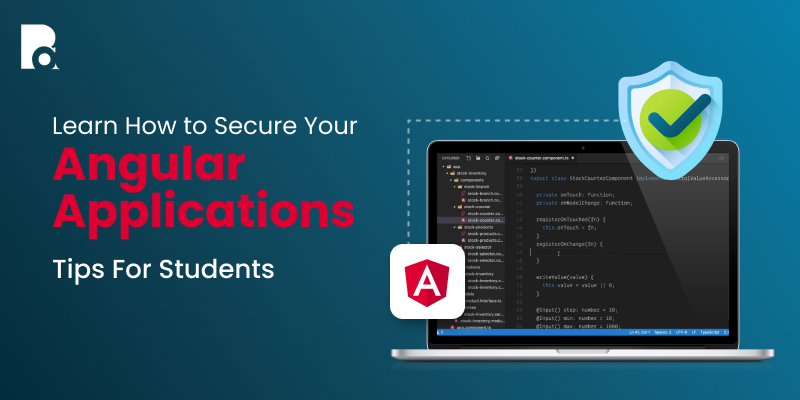
Learn How to Secure Your Angular Applications- Tips For Students
As giants like Sony, General Motors and Google use Angular for their websites and web apps, you can be sure that it is a robust framework. Developers need a platform that proactively neutralizes cyber threats. No one wants to build an app that is full of vulnerabilities that allow cybercriminals to break into servers or steal users’ data. Fortunately, the security in Angular is designed to proactively safeguard applications from a large number of threats.
Students who are new to the world of Angular need to learn the techniques and best practices to implement security in Angular applications. The information here is meant to guide students who are trying to develop Angular apps.
Types of Attacks on Angular Apps
- Cross-Site Scripting (XSS)
With malicious intent, attackers introduce harmful scripts into web app pages that are open on a user’s browser. They can hijack sessions, steal user data or spread malware.
- Cross-Site Request Forgery (CSRF)
Here, the malicious party will fool an authorized user of your app to perform an action within your app on the attacker’s behalf. They may trick them into revealing passwords or making money transfers.
- SQL Injection
This attack targets databases by adding malicious SQL commands into database queries and gaining access to the database. They can then corrupt the data, steal the data, delete it and much more.
- Clickjacking
In this scenario, attackers create transparent or opaque layers over a web page and add a webpage element like a button, ad or link (which seems legitimate but are actually not). Users unintentionally click on these harmful elements and have their data stolen or their webcam turned on or they may even download malware without meaning to do so.
- Man-in-the-Middle (MitM) Attack
Using eavesdropping techniques, this attack is designed to seize communication between the user and the web server while it is en route. The communication is captured and sometimes altered to suit the purpose of the attacker. Things like login credentials, credit card details and other confidential data can be stolen.
Learning How to Strengthen Angular Application Security
Does it make sense to create an attractive web app that functions smoothly but still has many security risks? Of course, it does not. Angular web developers need to learn how to make web applications that are not only visually appealing but are also robust and secure against various types of cyber attacks.
Some of the best practices and techniques for security in Angular include:
Authentication and authorization
- Use updated Angular version
The Angular version you use should be the latest one available. While this may seem obvious, many overlook this step. Go ahead and update the framework so you have the current improvements and security patches. The newest versions have all previous vulnerabilities and bugs are fixed.
- Apply solid password policies
The app you build should force users to create hard-to-guess passwords (combination of upper and lowercase letters, special characters, numbers etc.). This helps to block phishing attempts. Set the rules through a password strength functionality, enforcing strong and unique passwords with account lockout set-up for multiple incorrect tries.
- Use Multi-Factor Authentication (MFA)
App data is safer when MFA (Multi-Factor Authentication) is used. Suppose the user’s password is stolen, with MFA the perpetrator will still need to complete a further step (often, this involves a code sent to an email or phone number), without which they won’t get access. It’s a robust way to ensure that confidential and sensitive data remains safe.
- Hashing algorithms for user credentials
If a storage database gets hacked, any user password stored in plain text can be stolen. For this reason, using bcrypt, the passwords must be hashed and salted (this basically turns it into an unintelligible bunch of letters and numbers). Attackers will not be able to crack user credentials. You need to take these precautions to increase the security of the customer-facing web app you build .
Avoid exposing your API endpoints
When using your web app, users will expect that all their confidential data is protected and can only be accessed by them. As an Angular developer, you need to know the risk involved in exposing API endpoints. Think of these endpoints as your app’s data and functions. To make sure the communication between the frontend and backend of your web app is secure, keep your API endpoints well-documented, routinely maintained, and compliant to RESTful principles.
Data encryption
Things like user credentials, financial information, identity details etc. are sensitive data. Web apps developers need to ensure that data like this remains safe from unauthorized access. Any eavesdropping by outsiders can be prevented by using HTTPS (which is supported by Angular). So, even if the data in transit between client and server gets snatched, it will appear unreadable to the hackers. Similarly, data at rest needs to be encrypted so that your database is secure.
Cross-Site Scripting (XSS)
An attacker can steal user data or even take control of your app by slipping harmful scripts into your app. This occurs when user input is not checked and validated. Angular’s in-built DomSanitizer is helpful in this regard, as it cleans inputs and gets rid of any malicious code. Plus, {{expression}} binding in Angular increases app security by automatically escaping HTML (which means that it turns special characters in a web page’s text into safe versions)
Protection against Cross-Site Request Forgery (CSRF)
Attackers can trick genuine users into doing what they want within your web application. There are many ways in which they do this, but this should give you an idea of this type of attack. Suppose a user is logged into a bank website and also simultaneously has an unrelated website open on their browser (unknown to the user, the site may be malicious). Now, this malicious site may send a money transfer request to the user’s bank and, because he/she is already logged in, their bank will think the request is coming from their end and proceed to process it. So, for outgoing requests, it is vital that Angular web apps include special tokens which are unique to each session and protect your web app from such attacks.
Using Route Guards
Route Guards are guards of your webpages that check if a user has permission to enter a particular page (route) before letting them in. For example, based on pre-set conditions, it might check if the user is logged into a web app and only allow them permission to navigate the page. If not, it will redirect them to an error or login page. This ensures that only authorized users can access sensitive routes (admin page etc.) in your Angular web application.
Security Headers
In an attempt to prevent common attacks, HTTP response headers are used while developing Angular web apps. Angular’s HttpClient can be used to set HTTP headers, which are like special instructions that a web server sends back to the user’s browser when they visit a web app. For example, Content-Security-Policy (CSP) serves to block cross-site scripting (XSS) by defining which sources of content are safe to load. In the same way, Strict-Transport-Security (HSTS) informs the browser to always use a secure HTTPS connection for future visits to the site.
Input Validation and Sanitization
Attacks like SQL injection and XSS can be avoided when user inputs are validated and sanitized. Through 14 in-built validation rules, Angular’s form validation library only allows user inputs to be submitted to the server after it meets all the pre-defined criteria. Moreover, all potentially harmful elements are eliminated to sanitize the inputs. Basically, by default it considers all inputs as untrusted, until they pass the set criteria.
Secure Angular CLI Configuration
Angular CLI (Command Line Interface) is a command shell through which developers can efficiently build, update, test, deploy and maintain their web apps. But, they need to make sure it is configured securely. For this, you would need to strip down the features to the bare minimum required in the production environment. Passwords, secret keys or other sensitive data should not be exposed in the build configuration. It’s important to use the ‘ng update’ command to update Angular CLI to the latest patch version (run a global as well as local update). All this helps you maintain a secure development environment.
Secure Dependency Management
All the different pieces of code in each project are called dependencies and developers need to keep track of them and ensure that all are correct versions and updated. Security flaws in any of these dependencies (especially third-party code) leave your app project vulnerable to attacks. You can use npm (Node Package Manager) to effortlessly manage dependencies, also the npm audit tool serves to scan your project, find any flaws and helps you fix them.
Security Testing and Auditing
Without security testing and auditing, it is impossible to keep user data safe, find and fix security gaps and increase user trust. You can add ESLint to your Angular web app project to spot common vulnerabilities in your code. You would not have to run the code as this can be done in static mode. But, if you use OWASP ZAP or Burp Suite, you will have to run the code. Here, you will verify if your web app can withstand the simulated attacks that the tools create. Besides that, developers must audit their code and configurations regularly and check whether best practices are being used. This is not a one-time task, but an ongoing process.
Monitoring and Logging
If you do not use logging tools, your app may experience security incidents that you will be unaware of. Logging tools keep records of important events and user activities, while monitoring tools are meant to spot unusual app behavior. So, you are able to easily spot security threats and resolve them in real-time. Detailed logging and continual monitoring needs to be part of the application development cycle if you want to avoid security breaches and failures.
The process of securing an Angular application is an ongoing one, where particular attention is paid to every detail and continuous action is taken to make the web application secure. If you follow the best practices of Angular, you can manage to create a robust and secure application that will safeguard your users and their details. Angular developers should make the most of the opportunity to expand your knowledge on the latest security trends and implement all security measures available to them. Security is not a mere option — it is a critical part of the modern web development.
Emerging Web Development Security Threats That You Should Be Aware Of
- Supply chain attacks
These attacks are aimed at the libraries and tools used in your Angular app. In case one of the libraries or tools you rely on gets hacked, unknown malicious code may get injected into an application. That’s why third-party packages should be sourced from trusted sources and kept up-to-date to make sure known vulnerabilities are fixed.
- Advanced Persistent Threats
This involves targeted attacks executed over a long period with the intent to spy or steal information from a user. Such attacks are very sophisticated, and some of them may even stay hidden for years, turning out to be very dangerous. Regular security audits and monitoring for unusual activities are highly necessary for detecting and preventing APTs.
- Credential stuffing
It is when attackers attempt to log into user accounts, reusing lists of stolen usernames and passwords. In case users have duplicated passwords across different sites, this makes it very easy for attackers to break into accounts. This risk can be dramatically lessened by unique passwords and multi-factor authentication.
- Zero-day attacks
This occurs when the developer is not aware of a security flaw in their app and does not provide a fix or patch. Since there’s no immediate fix, attackers can quickly exploit these flaws. Keeping current with new vulnerabilities and applying patches as soon as they are available can minimize your risk of zero-day attacks.
How Can a Course Help You Learn Angular App Development?
To equip students with the skills necessary to cope in the real-world environment, these advanced angular courses contain case studies of security breaches. Through these, students would analyze the way in which the breaches occurred and what could have been done to prevent them. This helps students to recognize the various types of security vulnerabilities and the effect they can have in real-world scenarios.
It would cover particular techniques and best practices for security in Angular applications: properly sanitize user inputs so that no XSS attacks can get through, implement token-based authentication that prevents CSRF, and effectively use the built-in security features of Angular. Practical exercises can reinforce this learning, enabling students to try out what they have learned in a controlled environment.